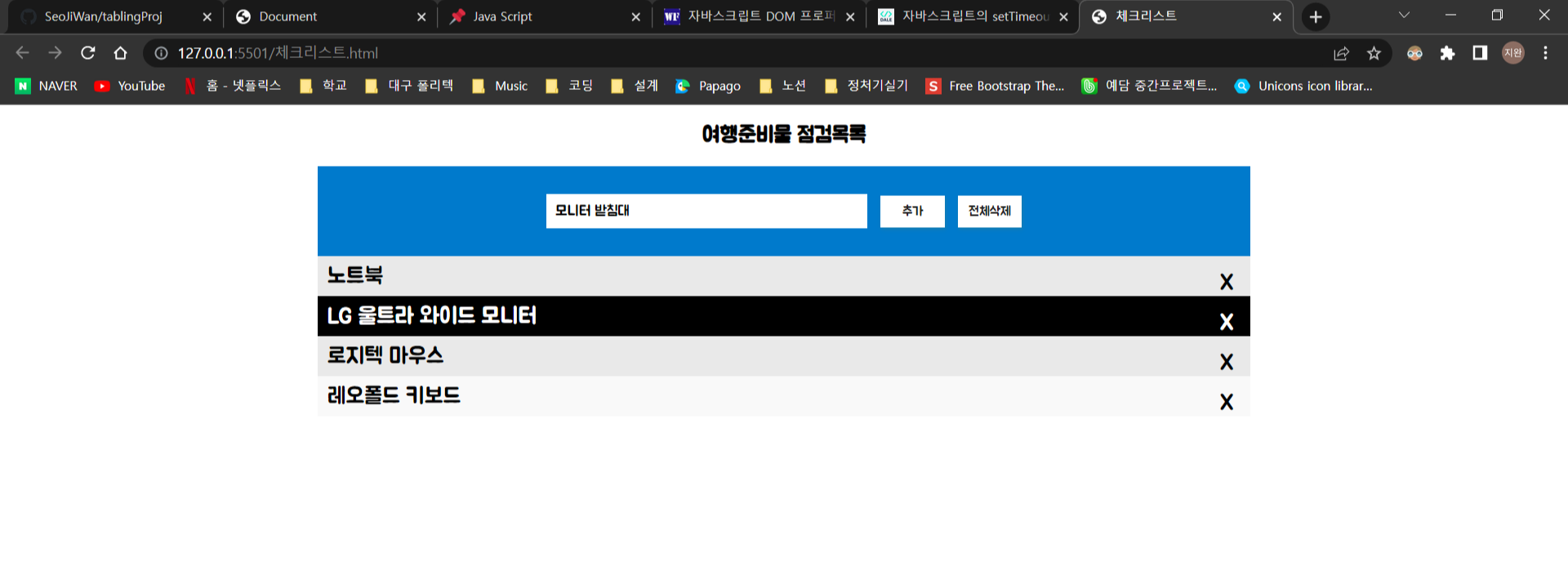
코드
html, javascript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>체크리스트</title>
<link rel="stylesheet" href="css/체크리스트.css" />
</head>
<body>
<div id="wrapper">
<h2>여행준비물 점검목록</h2>
<form>
<!-- <input hidden="hidden" /> -->
<input type="text" id="item" autofocus />
<button type="button" id="add" class="addBtn">추가</button>
<button type="button" id="remove_all" class="addBtn">전체삭제</button>
</form>
<div id="item_list"></div>
</div>
<script>
// 아이템들을 담을 아이템 리스트
let itemList = [];
// 아이템 중복확인 위한 변수
let dupl;
// 추가 버튼에 대한 이벤트
let addBtn = document.querySelector("#add");
addBtn.addEventListener("click", addList);
// 전체삭제 버튼에 대한 이벤트
let removeAllBtn = document.querySelector("#remove_all");
removeAllBtn.addEventListener("click", removeList);
// 엔터 입력시 이벤트 발생
document
.querySelector("#item")
.addEventListener("keypress", function (e) {
if (e.keyCode === 13) {
e.preventDefault();
addList();
}
});
// 아이템 추가 메서드
function addList() {
// 아이템 값 담음
let item = document.querySelector("#item").value;
// console.log(item);
// 중복체크 실행
checkDupl(item);
// 조건을 확인하고 아이템 리스트에 푸시
if (item != "" && dupl) {
itemList.push(item);
// console.log(itemList);
// 아이템 input 창 초기화
document.querySelector("#item").value = "";
document.querySelector("#item").focus();
// 아이템 리스트 출력
showList();
}
}
// 중복확인 메서드
function checkDupl(item) {
// 입력한 아이템이 아이템 리스트에 있는지 확인
if (itemList.includes(item)) {
alert("이미 담은 준비물입니다.");
document.querySelector("#item").value = "";
document.querySelector("#item").focus();
dupl = false;
} else {
dupl = true;
}
}
// 아이템리스트 출력 메서드 (테이블)
function showList() {
// 아이템 리스트를 for 문을 돌면서 테이블 태그로 생성
let list = "<table>";
for (let i = 0; i < itemList.length; i++) {
list += `<tr>
<td class="i">${itemList[i]}</td>
<td class="x" id="${i}">X</td>
</tr>`;
}
list += "</table>";
// 테이블 태그 출력
document.querySelector("#item_list").innerHTML = list;
// 아이템 리스트에서 삭제를 위한 X 태그들을 담음
let xMarks = document.querySelectorAll(".x");
for (let i = 0; i < xMarks.length; i++) {
// 여러 개 xMarks 중 유저가 xMark 를 선택하면 해당 xMark 에서 삭제 이벤트 발생
xMarks[i].addEventListener("click", removeOne);
}
}
// xMark 누르면 삭제 메서드
function removeOne() {
// getAttribute() -> 파라미터에 해당하는 속성을 가져옴
// this -> xMarks[i] 에서 선택되는 아이
let id = this.getAttribute("id");
// itemList 에서 해당 id 의 품목 삭제
itemList.splice(id, 1);
showList();
}
// 전체 삭제 메서드
function removeList() {
// 아이템 리스트 전체 삭제
itemList.splice(0, itemList.length);
showList();
}
</script>
</body>
</html>
css
@font-face {
font-family: bm;
src: url(../font/bamin/BMJUA_ttf.ttf);
}
* {
box-sizing: border-box;
font-family: bm;
}
#wrapper {
width: 60%;
margin: 0 auto;
}
h2 {
text-align: center;
}
form {
background-color: #007acc;
padding: 30px 40px;
text-align: center;
}
input {
border: none;
width: 350px;
padding: 10px;
font-size: 16px;
}
.addBtn {
padding: 10px;
width: 70px;
border: none;
background-color: #fff;
box-shadow: 1px 2px 4px #167dae;
color: #222;
text-align: center;
font-size: 14px;
cursor: pointer;
margin-left: 10px;
}
.addBtn:hover {
background-color: black;
color: white;
}
/* ul li 로 아이템 리스트 작업 */
ul {
margin: 0;
padding: 0;
list-style: none;
}
ul li {
cursor: pointer;
position: relative;
padding: 12px 8px 12px 40px;
background-color: #eee;
font-size: 18px;
}
ul li:nth-child(odd) {
background-color: #f9f9f9;
}
ul li:hover {
background-color: #ddd;
}
.close {
position: absolute;
right: 0;
top: 0;
padding: 12px 16px;
}
.close:hover {
background-color: #007acc;
color: white;
}
/* ul li 로 아이템 리스트 작업 끝 */
/* table 로 아이템 리스트 작업 */
table {
border-collapse: collapse;
/* border: 1px solid #000; */
width: 100%;
font-size: 25px;
background-color: #f9f9f9;
}
/* q - 이거 왜 따로 해야되지? */
tr:hover {
background-color: black;
color: white;
}
tr:nth-child(2n + 1) {
background-color: #e9e9e9;
}
tr:nth-child(2n + 1):hover {
background-color: black;
}
.i {
width: 95%;
padding-left: 10px;
}
.x:hover {
background-color: #007acc;
color: white;
}
.x {
text-align: center;
cursor: pointer;
padding-top: 15px;
}
/* table 로 아이템 리스트 작업 끝 */
실행화면
- Just Do It -
반응형
'JavaScript' 카테고리의 다른 글
[JavaScript] 문자열 (0) | 2022.07.20 |
---|---|
[JavaScript] 다양한 함수 선언 방법 (0) | 2022.07.20 |
[JavaScript] Date 객체 이용하여 기념일 계산하기 (0) | 2022.07.20 |
[JavaScript] setInterval 메서드 이용해 타이머 만들기 (0) | 2022.07.20 |
[JavaScript] 입력 폼에서 조건 체크하기 (0) | 2022.07.20 |